JavaScript Libs: Dinero.js is a library for working with monetary values
A Dinero object is an immutable data structure representing a specific monetary value. This means that you do not have to handle a currency value (like Dollar, Pound or Euro) as a floating point number but as an object. The object can be manipulated and formatted in a much safer way.
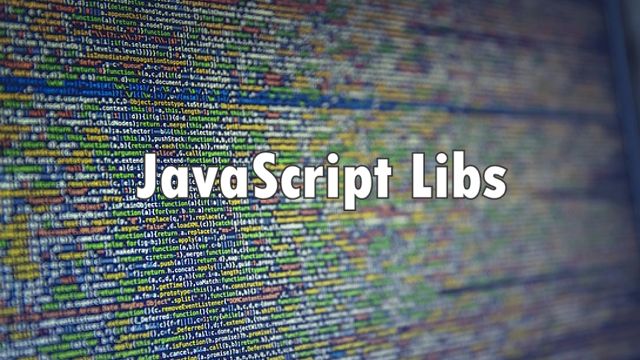
JavaScript Libs is a series of articles introducing useful JavaScript libraries to developers
Dinero.js
Dinero.js is a library for working with monetary values in JavaScript.
What it does
A Dinero object is an immutable data structure representing a specific monetary value. This means that you do not have to handle a currency value (like Dollar, Pound or Euro) as a floating point number but as an object. The object can be manipulated and formatted in a much safer way.
For example, you can’t accidentally add Dollars and Pounds, you can format the value correctly (with the correct currency symbol) and you don’t get the potential floating point maths errors.
A Dinero object holds the amount in minor currency units (such as cents or pence, e.g. USA 123 = $1.23), the currency as an ISO 4217 currency code and the precision, expressed as an integer, to represent the number of decimal places in the amount.
Creator: Sarah Dayan
License: Dinero.js is licensed under MIT.
Popularity: npm has about 500+ downloads per week.
Popular Functions
- Access: getAmount, getCurrency, getLocale and getPrecision.
- Manipulation: add, subtract, multiply, divide, percentage, allocate and convert.
- Testing: equalsTo, lessThan, lessThanOrEqual, greaterThan, greaterThanOrEqual, isZero, isPositive, isNegative, hasCents, hasSameCurrency and hasSameAmount.
- Configuration: setLocale.
- Conversion & Formatting: toFormat, toUnit, toRoundedUnit, toObject, convertPrecision and normalizePrecision.
Features
- Immutable and chainable API.
- Global settings support.
- Extended formatting and rounding options.
- Native Intl support (no additional locale files).
Where to Find Dinero
On npm: https://www.npmjs.com/package/dinero.js
On github: https://sarahdayan.github.io/dinero.js/
Recommended Installation:
– npm install dinero.js –save
– yarn add dinero.js
Examples:
const Dinero = require('dinero.js'); const price = Dinero({ amount: 234, currency: 'GBP' }); console.log('price is ' + price.toFormat('$0,0.00')); // £ 2.34 console.log('has pence: ' + price.hasCents()); // true console.log('value: ' + price.getAmount()); // 234 console.log('currency: ' + price.getCurrency()); // GBP //price.add(Dinero({ amount: 200 })); //-> error as we can only add the same currency var newPrice = price.add(Dinero({ amount: 123, currency: 'GBP' })); console.log('newPrice is ' + newPrice.toFormat('$0,0.00')); // £ 3.57. console.log('price is ' + price.toFormat('$0,0.00')); // £ 2.34 // Original price is unchanged as dinero is immutable
Further Examples:
How to Handle Monetary Values in JavaScript