How to shuffle a deck of cards for card games in Flutter/Dart
How to shuffle a deck of cards for card games in Flutter/Dart:
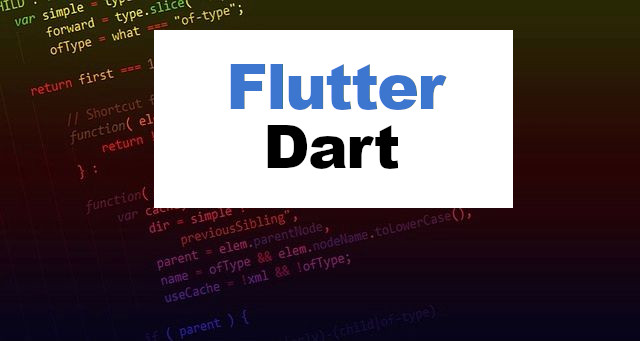
How to shuffle a deck of cards for card games in Flutter/Dart:
import 'dart:math'; void shuffleCards() { // create a deck of cards // we'll use a list of integer 0..51 to represent the deck // In practice define a PlayingCard class and make a list of that List<int> card = new List.filled(52, 0); // initialise deck of cards. Set the cards as 0-51 which can represent C2 to SA for (int i = 0; i < 52; i++) { card[i] = i; } // Fast deck-swap shuffle. Generate a random number and swap contents with that element // This way we'll make 52 swops only. It's good enough for card games. Random autoRand = new Random(); for (int i = 0; i < 52; i++) { int randomCard = autoRand.nextInt(52); int temp = card[i]; card[i] = card[randomCard]; card[randomCard] = temp; } // cards are now shuffled }